Production Grade Docker Compose
In this day and age, Docker is the status quo of building applications, allowing us to build and deploy applications using containers.
Docker is an open-source containerization technology utilizing the concept of containers.
There are dozens of benefits of using containerization technologies like docker including easy packaging and deployment of an application, easy collaboration between developers, easy isolation between systems, simple configuration patterns among many other advantages.
Now if you are a heavy docker user you will know that when building apps on top of docker we quickly run in to a problem, the problem is the management of several different containers quickly becomes complicated entangled.
Docker Compose is a tool that helps us overcome this problem and easily handle multiple containers at once.
version: "3.7"
services:
…
volumes:
…
networks:
…
Let’s see what these elements are.
Services
services refer to the basic containers’ configuration, let say we have an application dockerized and split into different images, so we will add each image as a separate service,
services:
frontend:
image: my-react-app
backend:
image: node-app
db:
image: Postgres
Volumes & Networks
In order to be able to save (persist) data and also to share data between containers, we use the volumes and networks. you can read more about them here.
Finally, now we have out of the way let us, deep-dive, into some tips and tricks for best practice and smart usage of the technology.
Best Practices
Making use of environment variables
The main advantage of using an external file for your environment variables is that you can keep said file out of your source control. After all, no one enjoys their passwords/API keys/other super-secret information being displayed all over the internet
After you have variables stored in your .env file you can use them directly in the docker-compose.yml file.
Using string interpolation (${string}) we can insert variables directly into our compose file.
version: ‘3.1’
services:
db:
container_name: Mongo-db
image: mongo:latest
restart: always
volumes:
- ./myData:/data/db
environment:
- MONGO_INITDB_DATABASE=${DATABASE}
- MONGODB_USER=${USERNAME}
- MONGODB_PASS=${PASS}
ports:
- 27020:27017
Defining your HEALTHCHECK in Docker Compose
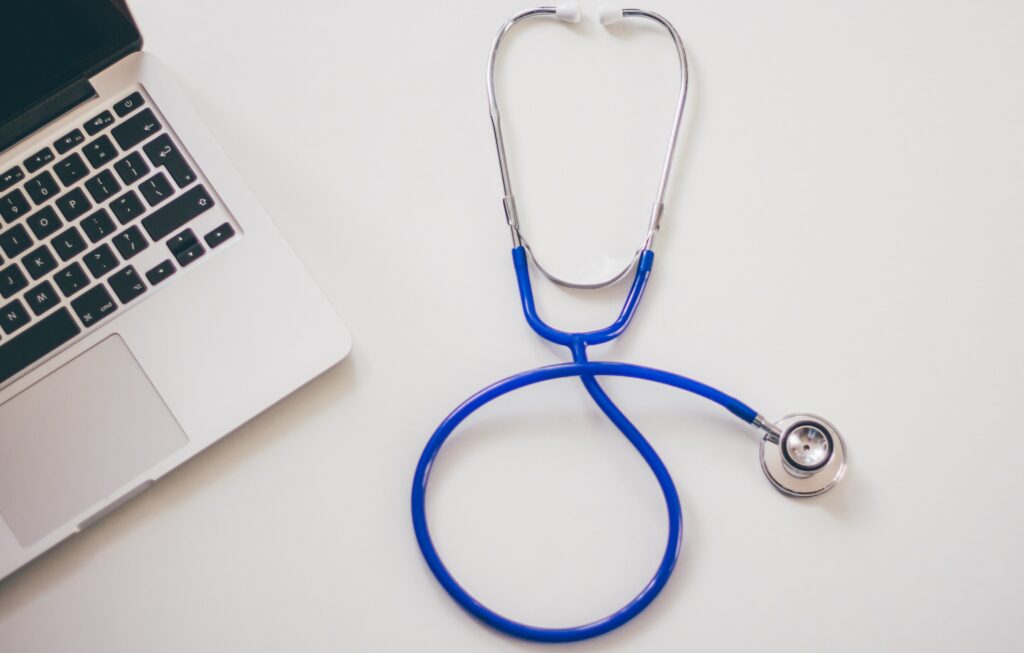
A Health Check means to check the health of any resource to determine whether that resource is operating normally. Here, it is about checking the health of Docker containers.
healthcheck:
test: "${DOCKER_WEB_HEALTHCHECK_TEST:-curl localhost:8000/test}"
interval: "60s"
timeout: "3s"
start_period: "5s"
retries: 3
Limiting CPU and memory resources of your containers:
web:
deploy:
resources:
limits:
cpus: "${DOCKER_WEB_CPUS:-0}"
memory: "${DOCKER_WEB_MEMORY:-0}"
If you set 0 then your services will use as many resources as they need which the default property.